In this post, I’ll be explaining the find and findIndex methods in Javascript programming, with the help of the Feynman technique and the world of Harry Potter. This is part of the Feynman Technique/20 hr Method challenge. For more information, click here.
In JavaScript, when it comes to arrays (grouping of information), it’s not unusual to have your eyes bug out due to the volume of information. When dealing with broken code, it’s not uncommon to feel indignant, after combing through it for hours, you realize you missed a “;”.
You are not alone, my friends.
What are the find and findIndex methods?
When it actually comes to finding stuff, as programmers, we’d rather run some code and have it figure it out automatically. Maybe having a remembrall and enchant it to tell you what you’ve forgotten would be the Harry Potter method, if we were all wizards.
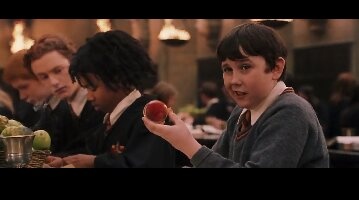
In the case of muggle programmers, we have the find method. We’ll use Neville Longbottom to show us how to use it in JS (and because I don’t know where I left my wand).
Find Method in Action
Say Dumbledore is looking for Neville Longbottom’s house points card. He’d have to look among all the other first years in Gryffindor house. If he were to have a muggle use Javascript, the card code may have been listed like this:
const student1 = {
student: `Harry Potter`,
housePoints: [-50, 60, 5, -5, 10],
};
const student2 = {
student: `Ron Weasley`,
housePoints: [-50, 50, -5, 10],
};
const student3 = {
student: `Hermione Granger`,
housePoints: [-50, 50, 10, -5],
};
const student4 = {
student: `Neville Longbottom`,
housePoints: [-50, 10],
};
const student5 = {
student: `Dean Thomas`,
housePoints: [5, -10, 15],
};
const student6 = {
student: `Seamus Finnigan`,
housePoints: [3],
};
const student7 = {
student: `Lavender Brown`,
housePoints: [2, -5],
};
const student8 = {
student: `Parvati Patil`,
housePoints: [10, 2, 15],
};
const gryffindor1stYrs = [
student1,
student2,
student3,
student4,
student5,
student6,
student7,
student8,
];
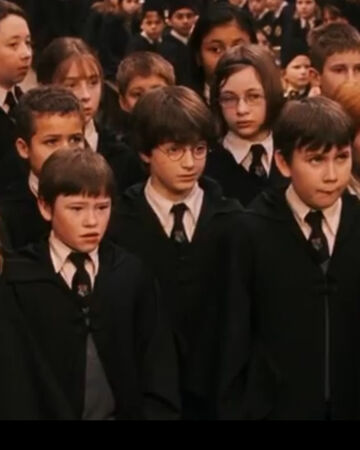
All the student cards are numbered from 1-8. Then they were grouped together under the group name gryffindor1stYrs. Dumbledore wants to award Neville 10 points as a special award. To find Neville’s card in the bunch, we’d have to use the following code, using the find method.
const specialAward = gryffindor1stYrs.find(
accio => accio.student === `Neville Longbottom`
);
console.log(specialAward);
What the what?!
Let me break this down…
- We can automatically pull Neville’s card out for next time, we named this group of instructions “specialAward”.
- In the instructions, we have the program go through the “gryffindor1stYears” bundle and “find” the following…
- We created an arrow function (which we explained back in our Uncle Roger Functions and Egg Fried Rice post) and called it “accio”.
- accio = gryffindor1stYrs.find, so it all breaks down into “gryffindor1stYrs, within the “student” entry, find `Neville Longbottom`”.
- To ensure we got it correct, the console.log(specialAward); comes back with Neville’s house points card.
{student: "Neville Longbottom", housePoints: Array(2)}
housePoints: (2) [-50, 10]
student: "Neville Longbottom"
__proto__: Object
Yep, that’s the one.
What About the findIndex Method?
Let’s assume Snape is in one of his moods and wants to deduct points from Harry’s card. Instead of wanting to find his card, he just wants to know where it is in the gryffindor1stYrs stack. Since that’s the case, we’d have to use the findIndex method to point that out. FindIndex instead of pulling the actual item you’re looking for, tells you where in the stack it is. So if we use the same information as we had listed above, all Snape would have to do is use the following js code:
const wheresPotter = gryffindor1stYrs.findIndex(
accio => accio.student === `Harry Potter`
);
console.log(wheresPotter);
Let’s explain what just happened:
- Snape created a new set of instructions called “wheresPotter”. This will him instant access to where Harry’s card is, whenever he wants to deduct more points from it.
- In that list of instructions, we go through the “gryffindor1stYrs” list and use the findIndex method. We run it through the arrow function.
- We’ve named the function “accio” again, which is the name for “gryffindor1stYrs.findIndex” (just like the find method, prior). Although we normally don’t give anything the same name in JavaScript (not like Java Programming), because both “accio”s will only live within their own function objects, they won’t affect anything outside of their code objects.
- We tell the program to match the student entry for “Harry Potter”.
- The console.log(wheresPotter) tells us where Harry’s card is located.
The system comes back with the number “0”.
Wait, Did it Work?
Before you freak out and say it doesn’t work, it actually did work. In Javascript, arrays start the first item at position 0, not position 1, and if we look back at the gryffindor1stYrs list, we see student1 is what would be position 0.
const gryffindor1stYrs = [
student1,
student2,
student3,
student4,
student5,
student6,
student7,
student8,
];
console.log(student1);
…and if we asked the system who student1’s card belong to, we get…
{student: "Harry Potter", housePoints: Array(5)}
…and it works! Who said you always need a wand to get things done?