In this post, I’ll be talking about the chain method in Javascript using the Feynman technique and the classic game of Monopoly. This post is part of the Feynman/20-hour challenge. For more information, click here.
Chain Keep Us Together
The chain method is a way where javascript sends information through a series of functions (instructions) and a final product comes out. Chains, unlike normal functions, usually work with arrays (blocks of numeric code, in this case) to end up with a product, and can keep on going, until the programmer (if they chose) ends it with a single value, in other words, turning the array into a single item (if capped off with the reduce method).
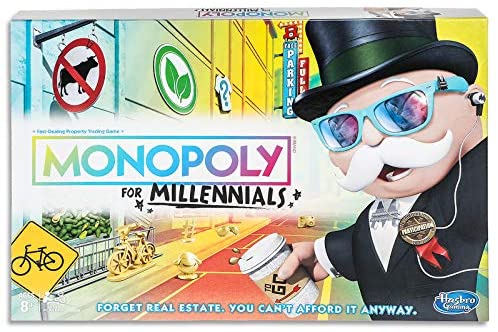
How Does the Chain Method Work?
If we decided to play a game of Monopoly, instead of counting how much money and property someone has to win, we see who is the boss by using the following rules:
- Only count the property owned + houses and hotels, and cash remaining (non-mortgaged)
- charge income tax on whatever is left (10%)
- and then use the average (only on what’s counted) to see who wins.
Yeah, we can get pretty brutal.
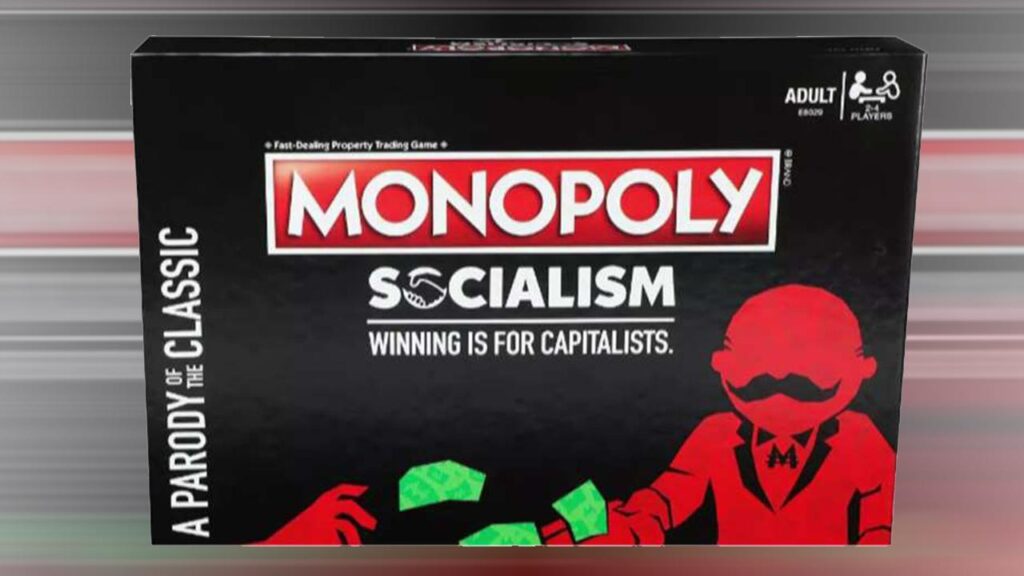
If we were to break down what we wanted to do with the chain method, it would look like this:
1 const incomeTax = 0.1;
2 const playerScore = property =>
3 property
4 .filter(property => property > 0)
5 .map(property => property - property * incomeTax)
6 .reduce((acc, property, i, arr) => acc + property / arr.length, 0);
Translation of the Code:
Line 1: Assigned name for the “incomeTax” at 10%.
Lines 2-6: Arrow function (covered in our JavaScript Functions post.
Below are the instructions to javascript what the series of rules and events are for our chain method function” playerScore”. The property array that’s entered will go through the following fiery hoops:
- filter the property elements (items) and only take the ones greater than 0 (which means all mortgaged properties will not be counted).
- (map) – take the info that passed through the filter and take the income tax out (property – (property * incomeTax))
- whatever is left, add together and divide it by the length of the array.
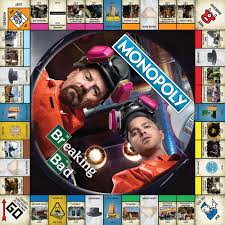
Granted, we’re not talking Heisenberg level of gameplay, but we’re not talking about any slouches either.
…So Who Won?
So if we put our data into this code and ask JavaScript to tell us who won:
const topHat = playerScore([60, 240, 340, 260, 200, 200, -200, 150, 150, 1620]);
const dog = playerScore([100, 180, 180, 700, 500, 1320, -200, 1250]);
const car = playerScore([-60, -120, 260, 560, 880, 400, 500]);
const thimble = playerScore([100, 200, 370, 370, 390, -350, 950]);
const players = [topHat, dog, car, thimble];
console.log(players);
We get the following response:
(4) [322, 543.8571428571429, 468, 357]
The dog hosed us all.
Here’s the final scores:
- (try again) topHat = 322
- (1st place) dog = 543 (forget the decimal points – he doesn’t need them)
- (2nd place) car = 468
- (3rd place) thimble = 357
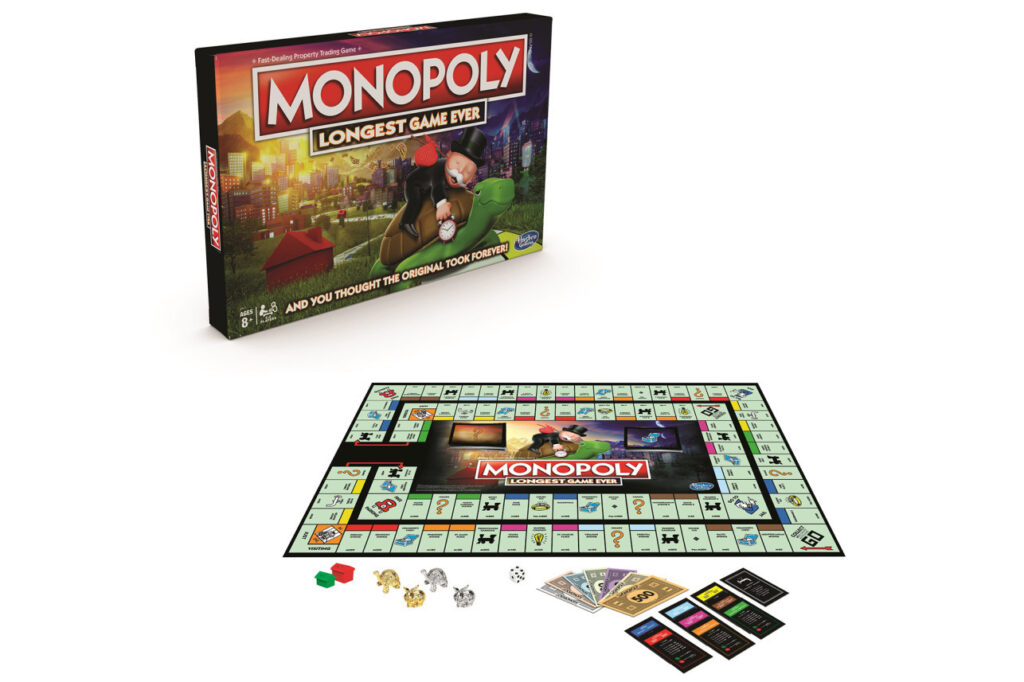
How Did The Dog Win?
If you want to check the math, here we go:
- topHat – If you only add his non-mortgaged properties and his leftover cash, you’d come out to 3220 – 322(tax) = 2898/9 (counted properties+ cash)= 322
- dog – Cash value of everything non-mortgaged is 4230 – 423(tax) = 3807/7 (counted properties + cash)=543.857…
- car – Cash value = 2600 – 260 (tax) = 2340/5 = 468
- thimble – cash value 2380 -238 = 2142/6 = 357
Although topHap had enough cash to secure 2nd place, if we went by the old route, he ended up in the last place by these rules.
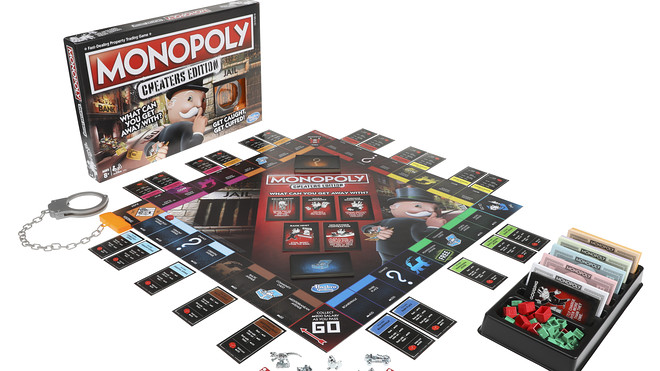
Last Man Standing
You can keep adding new chains into the function as long as the output from each (for the exception of the last), still produce an array. The last function can produce a single value, but no other function can be added, once that’s done.
Chains can be used in place of loops, however, it’s generally not a good idea to add any methods that will permanently change the parent array-like splice or reverse, as it may cause more headaches down the road, the longer your code becomes. It’s also a good idea if you boil down your coding as much as you can since no one wants to go through a hot mess of code that they can’t read.
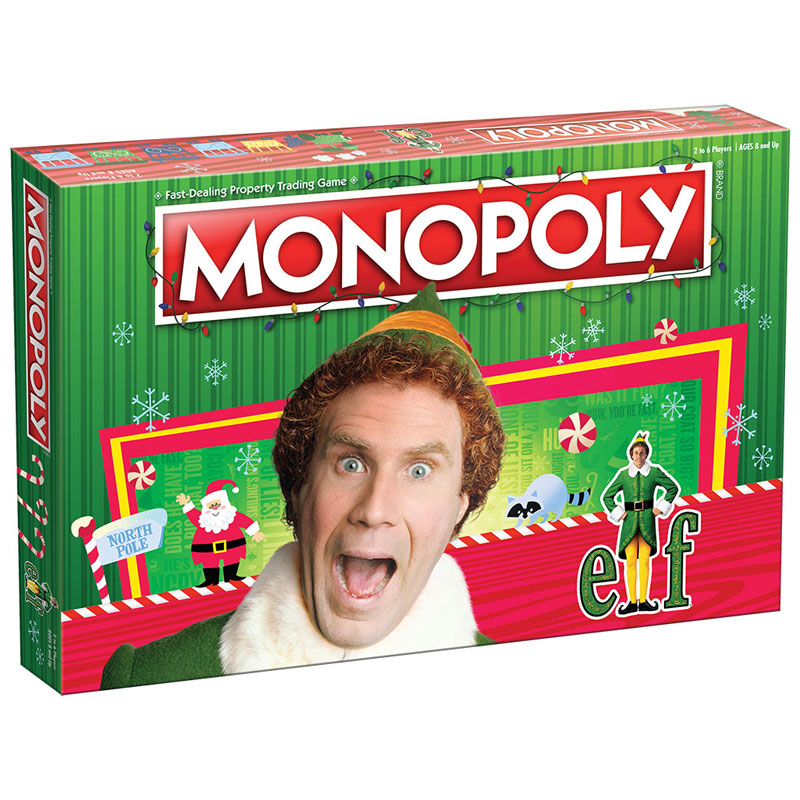
Anyway, happy coding and enjoy the holidays. ๐
Want More About JavaScript Chain Method?
Check out the links below for more info: