Today’s post is on the JavaScript: Filter Method explained using the Feynman Technique and is part of my Feynman Technique/20 hr Method challenge. For more information, click here.
When dealing with a group of numbers that’s tied together (which in JavaScript we called an “array“), we can set up a request for JavaScript to go through that group of numbers and filter out (using the Filter Method) what we don’t want, creating a new array with the numbers that we want to keep.
For instance, here’s a what an array would look like in code:
const movements = [200, 450, -400, 3000, -650, 130, 70, 1300];
Here’s the anatomy of the code above:
- const = constant (this means that the info listed in the following name won’t change. There can be multiple “const” in a code, but with different names. Think of it as a box of stuff stacked in a specific order.
- “movements” – this is the name of this box. Each const will only have one name and cannot have another with the same name. Think of the name of the box of stuff like “kitchen” or “bathroom” if you were moving.
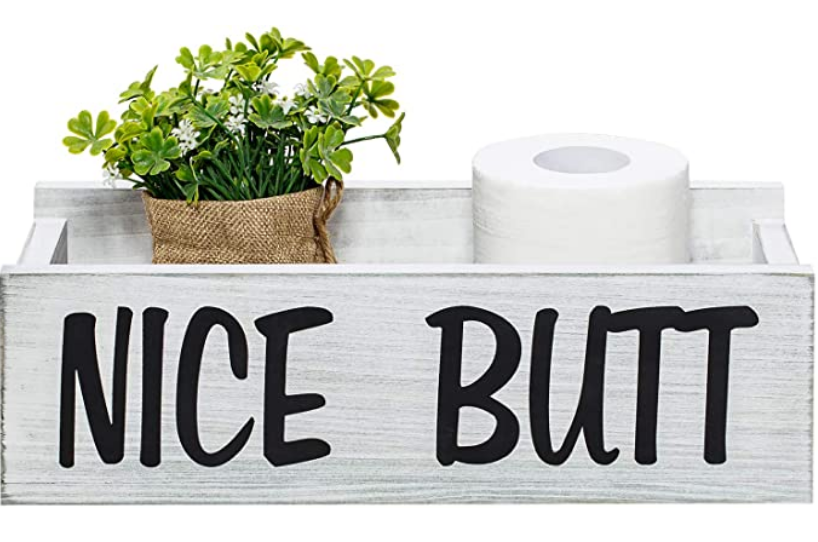
- “=” is the same here as it is in math.
- [ ] = anything in these brackets is called an array, which is a grouping under one const name, in a specific order, however, each item (called “element”) within these brackets still keeps its own value. In other words, a “1, -1” are still considered two different items in this box.
How can we use the Filter Method to filter stuff in the box?
If we wanted to take out all the negative numbers in the box “movements” and put them into a new box, we’d have to do the following:
const deposits = movements.filter(function(mov) {
return mov > 0;
});
We created a new box named “deposits”, taking the box named “movements” and told Javascript to filter it, using the directions in the function. Here’s an easier breakdown:
- Deposits = new box name
- movements.filter = we are taking the group of numbers and passing it through a filter. How we will filter it will depend on the information in the function, which is…
- function = our way to telling the program “after this is the list of instructions on what to do.
- (mov) = This is called the “argument”. It’s the info that you will need to work through the instructions in the above function. In here, it’s the group of numbers that’s inside the box we called “movements”. How do we know this? Because movements.filter told us to filter the stuff inside the movement’s box. The argument only lives in the function and doesn’t exist outside of it. Think of it like those extra pieces of cardboard in a box to create smaller boxes, but if you try to pull it out, it’ll just end up being a folded piece of cardboard.
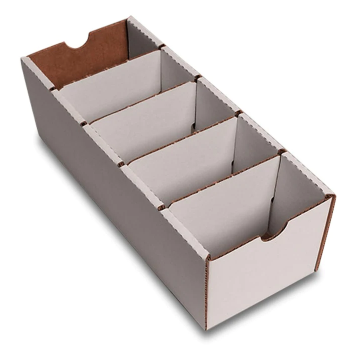
- return = this is considered the final answer to this instruction.
- “mov > 0” = in this case of the list of instructions, this is what we are asking the program to do. We are saying to compare each item in the movement’s box and if they are less than “0”, then don’t include them in the new group.
The result of this is:
(5) [200, 450, 300, 70, 1300]
…which is all the positive numbers from the “movements” group.
How about if we want to take out all the negatives?
If we wanted to take out the negative numbers, we can do it this way:
const withdrawals = movements.filter(mov => mov < 0);
This is another way of putting together a function (list of instructions). Let me break it down with the new info:
- withdrawals = this is the new name of our box.
- (mov => mov < 0); = This is us telling Javascript to use the stuff in the movements box, as we did before, and use it in the instructions, which in this case is saying “if the individual number in the movements’ box is less than “0”, then add it into the withdrawal’s box.
(3) [-400, -650, -130]
…this is what’s left after all the numbers in the “movements” box went through the instructions.
Pingback: JavaScript: (DOM) eventListener & Loops | Janifer